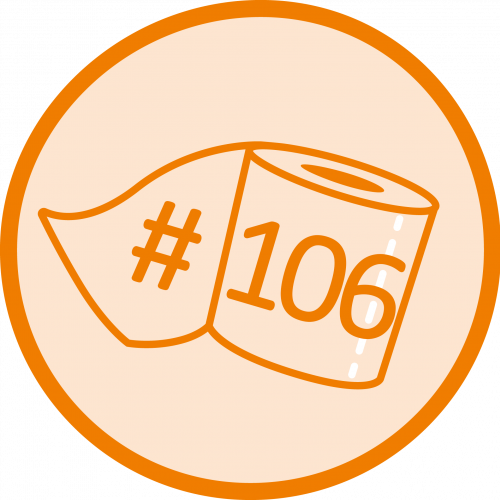
Before I get stuck, I’d rather use MapStruct
Problem
An unpopular and error-prone task, which is recurring especially in multi-layered architectures, is the writing of mapper classes for similar objects, e.g. between database entities and request / response DTOs. Writing unit tests for such mappers is also cumbersome.
Solution
The Java library MapStruct (http://mapstruct.org) takes on this task by generating mapping code automatically, based on annotations.
Advantages:
- Generation at compile time: no influence on performance
- Generated code uses getter / setter methods, so it's type safe, easy to debug and to understand / extend
- Reasonable default conventions, behavior can easily be overwritten
Example
Two complementary classes (getter / setter methods not shown):
public class CupOfCoffee {
private Enums.Brand brand;
private double temperature;
private int volume;
private double milk;
private double sugar;
private boolean isCorretto;
}
public class CupOfCoffeeDTO {
private String brand;
private String temperature;
private int volumeOfCup;
private double milk;
private double sugar;
private boolean isCorretto;
}
MapStruct base class:
@Mapper(componentModel = "spring")
public abstract class CoffeeMapper {
@Mapping(source = "volume", target = "volumeOfCup")
@Mapping(source = "temperature", target="temperature", numberFormat = "$#.0")
public abstract CupOfCoffeeDTO toCupOfCoffeeDTO(CupOfCoffee entity);
@InheritInverseConfiguration
public abstract CupOfCoffee toCupOfCoffee(CupOfCoffeeDTO dto);
}
While compiling, MapStruct generates the mapping code according to the annotations. The explicit @Mapping annotations are only needed for non-trivial mappings (such as different variable names, number formats, etc.). The abstract class can still be extended by explicit mapping functions.
Caution:
- You have to recompile after each change of the abstract mapper class
- Sometimes does not work very well with Lombok (another annotation-based code generator)
Further Aspects
---
Author
Magnus S. / Software Architect / Business Division Automotive Bavaria
Click here for the Toilet Paper #106: Before I get stuck, I’d rather use MapStruct (pdf)