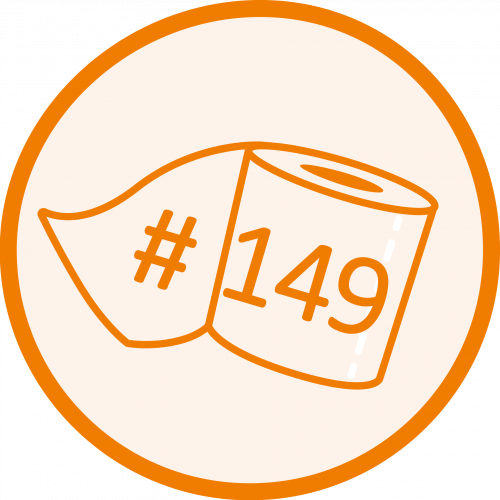
Testing with Mockito
Problem
With its possibilities to mock classes, Mockito is a very powerful tool. But when the equals() methods of the objects to be checked do not exist or are faulty, Mockito reaches its limits with its verify() methods. Normally, the classes are simply fixed and that’s it. In the case of external classes, it’s not that simple. There are several possibilities: transferring them into their own classes, hiding them very well in a wrapper or switching straight to Kotlin.
Solution
A useful solution to completely test the code is to use ArgumentCapture. This makes it possible to capture the instances during verify() and check them later.
Example
1 FuelPortion expectedFuelPortion = FuelPortion.DYNAMIC;
2
3 Engine myMockEngine = mock(Engine.class); //Or with @Mock
4 Car myTestCar = new Car(myMockEngine);
5
6 myTestCar.startEngine();
7
8 ArgumentCaptor<EngineConfiguration> engineConfigurationCapture = ArgumentCaptor.forClass(EngineConfiguration.class); //Or with @Captor
9 verify(myMockEngine).start(engineConfigurationCapture.capture()); // capture() is the central call for ArgumentCapture
10
11 EngineConfiguration actuelEngineConfiguration = engineConfigurationCapture.getValue();
12 Assert.assertEquals(expectedFuelPortion, actuelEngineConfiguration.getFuelPortion());
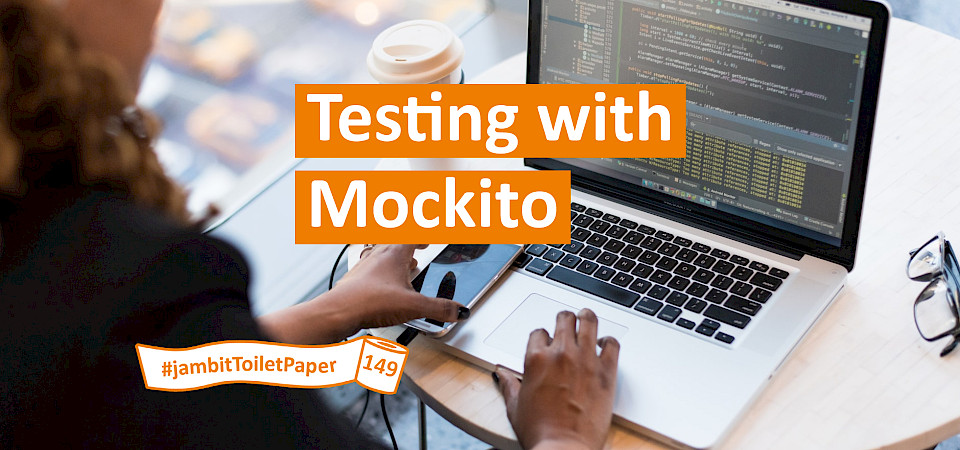
Further Aspects
- https://www.vogella.com/tutorials/Mockito/article.html
- https://www.baeldung.com/mockito-series
- https://github.com/mockito/mockito
---
Author: Alexander Pöhlmann / Software Engineer / Office Leipzig
Download Toilet Paper #149: Testing with Mockito (PDF)
Want to write the next ToiletPaper? Apply at jambit!