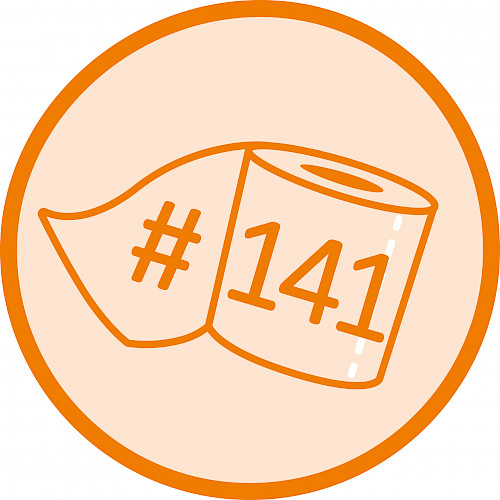
Interactive forms with Formik
Problem
Interactive forms should meet the following requirements:
- Input fields should be validated.
- The user should get feedback.
- The submit button could be disabled while the form is submitted.
Of course, we can implement these requirements using React alone. But for that, we would need to write a lot of code in React. We could abstract a lot of things, but why reinventing the wheel?
Solution
Formik help us developing our forms by taking care of recurring tasks (e.g. form state, validation, error handling).
It is a popular library and mentioned in React's documentation as a fully-fledged solution – see below. It has a clear API and is very easy to integrate into projects.
Example
const formSchema = Yup.object({
username: Yup.string().min(2, "At least 2 characters").required("Required"),
});
type FormValues = Yup.InferType<typeof formSchema>;
type Props = { initialValues: FormValues };
export const FormikForm = ({ initialValues }: Props) => {
const formik = useFormik<FormValues>({
initialValues,
validationSchema: formSchema,
onSubmit: (v: FormValues) => { setTimeout(() => setSubmitting(false), 900); }
});
const { isSubmitting, errors, touched, values, setSubmitting,
handleBlur, handleChange, handleSubmit } = formik;
return (
<form onSubmit={handleSubmit}>
<label>
Username
<input name="username"
value={values.username}
onBlur={handleBlur}
onChange={handleChange} />
</label>
{touched.username && errors.username && (
<span aria-live="polite">{errors.username}</span>)}
<button type="submit" disabled={isSubmitting}>Send</button>
</form>
);
};
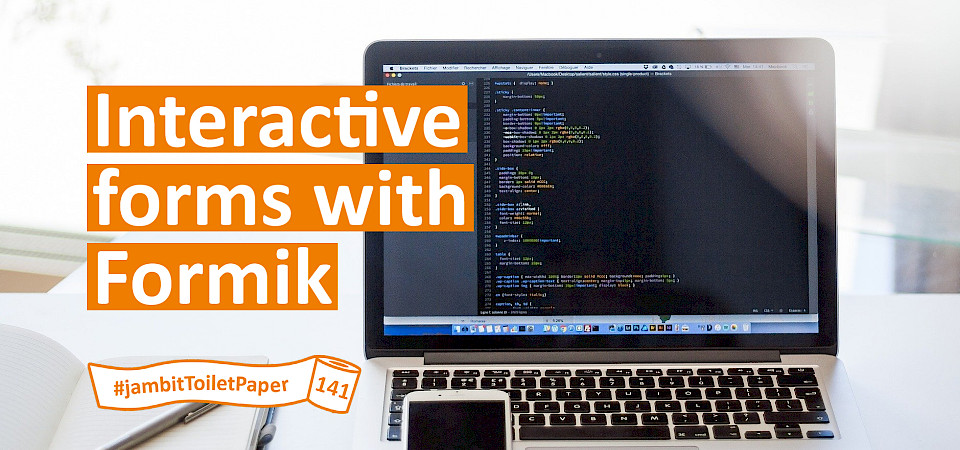
Further Aspects
- https://formik.org/docs/overview – Formik documentation
- https://reactjs.org/docs/forms.html – Formik as a fully-fledged solution
---
Author: Kemal Kaya / Software Engineer / Business Division New Business
Download Toilet Paper #141: Interactive forms with Formik (pdf)
Want to write the next ToiletPaper? Apply at jambit!